Java Syntax
A Java program consists of one or more classes one of them must be public and must have a method with the following signature:
​
public static void main (String[] args).
​
Basically, the main() method will instantiate appropriate objects and send them "messages" (by calling their methods) to perform the desired tasks. The main() method should not contain any complicated program logic nor program flow control.
Identifiers
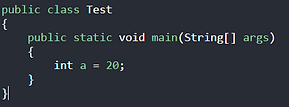
Identifiers are unique names assigned to things such as classes, variables, and functions across the program.
​
They may contain:
​
-
Underscore( _ ) and dollar($) (only special characters allowed in naming identifiers.)
-
Unicode characters such as numbers, and alphabets.
​
In the above Java code, we have 5 identifiers namely :
​
Test : class name.
main : method name.
String : predefined class name.
args : variable name.
a : variable name.
​
Literals
These are the identifiers that have inherent worth. These can be used to create variables. Additionally, literals can be thought of as constants.
Literals come in a variety of formats, including numeric, character, and string.
​
​
​ a. Numeric Literals - This has 4 kinds of variations:
1. Decimal - Any number of base 10 (such as 87.53)
​
2. Binary - Any number of base 2 (such as 11011, 11000)
​
3. Octal - Any number of base 8 (such as 1177)
​
4. Hexadecimal - Any number of base 16 (such as A54C)
​
​
b. Floating point Literals - We can only express numeric numbers using a decimal point (.). These are fractional numbers that cannot be represented in full integer form.
​
For instance: 10.876
​
c. Character Literals - These are the literals that deal with characters, that is, with inputs that are not of the type numeric.
​
1. Single quoted character - This encloses all characters with a single length within single quotes.
For instance: 'a','j'.
​
2. Escape Sequences - These are the characters preceding by a backslash that when printed on screen perform a specific function, such as producing a tab or a new line.
Example: ’\n’
Keywords
Keywords are unique identifiers assigned by the compiler. These are not permitted to be used to name variables, classes, or functions. These are protected terms.
​
Some of the keywords are:
​
-
Abstract – This keyword specifies that the class is an abstract class.
-
Boolean – This is a data type specifier which mentions that a particular variable is boolean.
-
Byte – this is a data type specifier which specifies a particular variable to be of byte type.
-
Case – a switch case keyword which specifies program to be performed if a particular case is satisfied.
-
Catch – during a throw case of error handling catch encloses actions to be performed if exception occurs.
-
Break – The break keyword breaks the control out of a loop.
-
Void – this keyword renders a method non-returnable.
-
Char – This is a data type specifier which specifies that the variable is of character type.
-
Class – This keyword specifies the creation of a new class followed by a class name.
-
Extends – This is used to indicate that the class mentioned after it is the derivation of a superclass.
Comments
Comments are required if the developer want to provide documentation to a program's declared function.
This is to improve the readability and comprehension of the code. The compiler does not execute comments and they are simply ignored during execution.
Comments come in a variety of types:
​
​ a. Single Line Comments - This type consist of a single line of comment generally written after a code line to explain is meaning.

​ b. Multi Line Comments - These comments span for multiple lines throughout the codebase. They are generally written at the beginning of the program to elaborate about the algorithm.
These are also used by developers to comment out blocks of code during debugging. They comprise of a starting tag(/*) and an ending tag(*/)
​
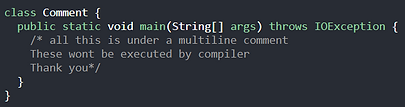
​ c. Documentation Comments - Typically, documentation comments are utilized while writing large programs for a project or software application, as they aid in the creation of documentation APIs.
These APIs are required for reference purposes, such as to determine which classes, methods, and arguments are utilized in the code. The Javadoc tool processes these comments while generating documentation.